FREE Bachelor of Computer Engineering C Language Questions and Answers
Which C expression is legitimate?
A variable name cannot contain a space, comma, or the symbol $.
What will the following C code produce as its output?
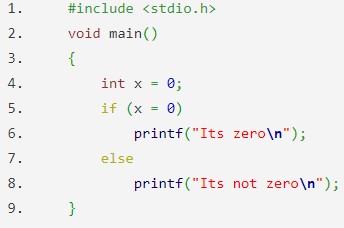
The variable x is given a value of zero in the C code above. We are giving x a new value of zero in the if condition. Keep in mind that we are "NOT" comparing the values to zero (you can see that there is only one "=" symbol, not two "==" signs). As a result, the else condition's printf() function is called, causing "Its not zero" to be shown and the if condition to become false.
What one of the following is untrue?
If a variable is declared but not defined, it is not a mistake. Extern declarations, for instance.
The ________ symbol is used to specify the C-preprocessors.
The # sign is used to specify C-preprocessors.
Which keyword is used in a C program to stop a variable from changing at all?
In a C program, the keyword constant is const.
What will the following C expression's value be? (x = foo()) ! = 1 assuming that foo() yields 2
The sub-expression "x = foo()" in the C programming language will give the variable "x" a value of 2. The result will be 1 as a result of the subsequent check to see whether this value is not equal to 1.
What will the following C code produce as its output?
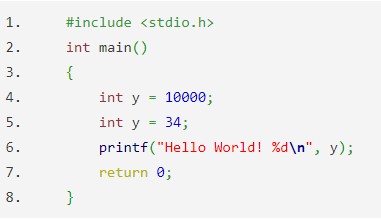
Redefining y causes an error because it has already been defined.
Output:
$ cc pgm2.c
pgm2.
c: In function ‘main’:
pgm2.
c:5: error: redefinition of ‘y’
pgm2.
c:4: note: previous definition of ‘y’ was here
Who is the C language's father?
The father of the C programming language is Dennis Ritchie. At the United States' American Telephone & Telegraph Bell Laboratories, the C programming language was created in 1972.
Which of the following is a name for a C variable that is invalid?
An error occurs because only underscore and no other special characters are permitted in variable names.
In the header file, there is a predefined function called scanf().
The "stdio.h" header file has a predefined function called scanf(). Input and output operations are performed by printf and scanf() in C. The header file stdio.h contains these function statements.
Which keyword is used in a C program to stop a variable from changing at all?
In a C program, the keyword constant is const.
Which statement regarding variable names in C is accurate?
It cannot begin with a digit, per the convention for C variable names.
Which of the following declarations does the C language not support?
In Java it is acceptable, but in C it is not.
What is the name of the C property that enables the creation of several executables for various platforms?
The preprocessor feature known as conditional compilation allows for the creation of various executables.
What will the following C code produce as its output?
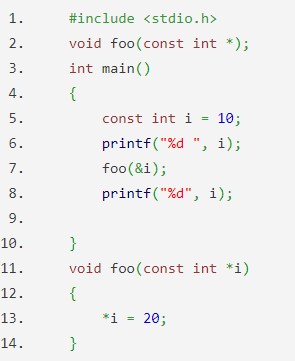
A const type value cannot be altered.
Output:
$ cc pgm1.c
pgm1.c: In function ‘foo’:
pgm1.c:13: error: assignment of read-only location ‘*i’
Which conversion type is not permitted?
A float cannot be converted to a pointer type.